Step-by-Step tutorial to build an android application which reads text from an image like OCR, using Google Vision API
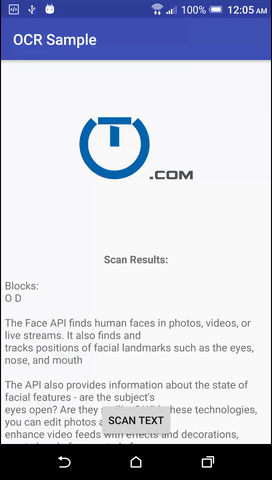
Step 1:- Create a new Project.
Step 2:- Add the following dependency in module-level
build.gradle file:-
compile 'com.google.android.gms:play-services-vision:9.8.0'
Step 3:- Set the style in styles.xml present in values
folder of res folder,
style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar"
Step 4:- Add meta-data to AndroidManifest.xml,
<meta-data
android:name="com.google.android.gms.vision.DEPENDENCIES"
android:value="orc"/>
android:name="com.google.android.gms.vision.DEPENDENCIES"
android:value="orc"/>
Step 5:- Now Design the layout in activity_main.xml,
<?xml
version="1.0" encoding="utf-8"?>
<ScrollView
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:android="http://schemas.android.com/apk/res/android" >
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.himalayabhagwani.textrecognitionimage.MainActivity">
<ImageView
android:layout_width="match_parent"
android:layout_height="400dp"
android:id="@+id/image_view"
android:scaleType="centerInside"
android:layout_alignParentTop="true" />
<Button
android:layout_width="match_parent"
android:layout_below="@+id/image_view"
android:layout_marginTop="15dp"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:id="@+id/btnProcess"
android:scaleType="centerInside"
android:text="PROCESS"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/txtResult"
android:text="No Text"
android:textSize="24sp"
android:gravity="center"
android:layout_below="@+id/btnProcess"
android:layout_centerHorizontal="true" />
</RelativeLayout>
</ScrollView>
<ScrollView
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:android="http://schemas.android.com/apk/res/android" >
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.himalayabhagwani.textrecognitionimage.MainActivity">
<ImageView
android:layout_width="match_parent"
android:layout_height="400dp"
android:id="@+id/image_view"
android:scaleType="centerInside"
android:layout_alignParentTop="true" />
<Button
android:layout_width="match_parent"
android:layout_below="@+id/image_view"
android:layout_marginTop="15dp"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:id="@+id/btnProcess"
android:scaleType="centerInside"
android:text="PROCESS"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/txtResult"
android:text="No Text"
android:textSize="24sp"
android:gravity="center"
android:layout_below="@+id/btnProcess"
android:layout_centerHorizontal="true" />
</RelativeLayout>
</ScrollView>
Step 6:- Now write the code in MainActivity.java,
import android.graphics.Bitmap;import android.graphics.BitmapFactory;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.util.SparseArray;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import com.google.android.gms.vision.Frame;
import com.google.android.gms.vision.text.TextBlock;
import com.google.android.gms.vision.text.TextRecognizer;
public class MainActivity extends AppCompatActivity {
ImageView imageView;
Button btnProcess;
TextView txtResult;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageView = (ImageView) findViewById(R.id.image_view);
btnProcess = (Button) findViewById(R.id.btnProcess);
txtResult = (TextView) findViewById(R.id.txtResult);
final Bitmap bitmap = BitmapFactory.decodeResource(getApplicationContext().getResources(),R.drawable.imp);
imageView.setImageBitmap(bitmap);
btnProcess.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
TextRecognizer textRecognizer = new TextRecognizer.Builder(getApplicationContext()).build();
if (!textRecognizer.isOperational())
Log.e("ERROR","Detector dependencies are not yet available");
else {
Frame frame = new Frame.Builder().setBitmap(bitmap).build();
SparseArray<TextBlock> items = textRecognizer.detect(frame);
StringBuilder stringBuilder = new StringBuilder();
for(int i=0;i<items.size();i++)
{
TextBlock item = items.valueAt(i);
stringBuilder.append(item.getValue());
stringBuilder.append("\n");
}
txtResult.setText(stringBuilder.toString());
}
}
});
}
}
Suppose I have an Indian currency, there are around twenty different scripts there. I just want to have English content and put each different phrases like "Reserve Bank of India", Guaranteed by central government", "I Promises to pay the bearer the sum of twenty rupees, "governor's name" and any other numerals like 10, 20, 50 100 or 500 into different varaibles and post it to a remote server. How do I do it?
ReplyDeleteI am working on a project to help farmers and biggest challenge is that they cannot read or write, I need to have a system to get their soil health card details when they show to the camera from within the app.
You have given me a good hope. I am a learner. Please guide me through.
http://stathunt.blogspot.com/2018/08/step-by-step-tutorial-to-make-android.html
Delete